The Third Building Block that we will talk about continues to build on the previous two. We have given our users the ability to arbitrarily add customer specific data and logic to our application. Now, we will also allow our users to take action within our application at certain key moments.
The third building block we are discussing is called the Rule System. For programmers, this is equivalent to a “pub sub” system in programming languages. For web developers, the most common example you would be familiar with is working with Javascript.
Take a look at this example. We are waiting for an event called “load” from the body of the HTML document, and when this happens we want the function to be executed. As you’re probably aware, in javascript you can add as many functions as you would like to be executed when the document body loads.
In this example, we have one “publisher”, which is the body who would broadcast the event “load” at the right time. We also have many “subscribers”, which are actions that happen when the event is broadcasted. This is an extremely important concept in programming, and exactly what we’ll be modeling with the Rule system so that configuration users can take advantage of this third tool.
Let’s take another look at an example Rule system using Zendesk’s as our example.
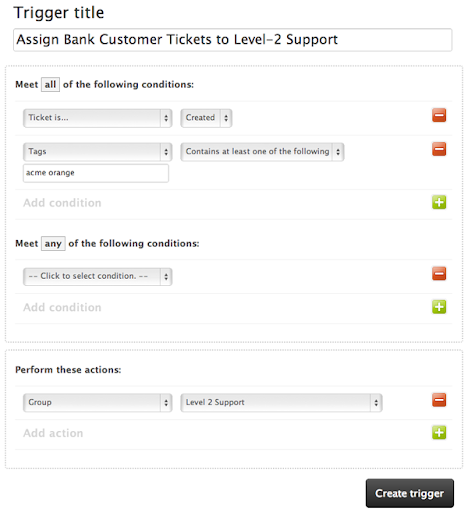
You can, right off the bat, see how we are reusing the Custom Field and Condition System. We are now adding two more elements. We are adding a “Trigger”, which is our event that will be broadcasted within the application. We are also adding an “Action” to configure what should be done when the event is broadcasted.
Let’s take a look at an example. Let’s say that our customer wants a custom email to be sent to all new users that sign up to the application if the user’s favorite color is blue. Thinking back to the last two chapters, first we’ll create a new Custom Field to store the user’s favorite color, and then we’ll set up a condition that compares their favorite color to “blue”.
Now, let’s create our rule. In pseudo code, our logic will basically say this:
When a user is created, if conditions are true then send custom email
In our codebase, let’s look at an example of how you might trigger this rule in the User model:
This is basically our application broadcasting “A user has just been created” message. A rule that matches the “user is created” trigger, would then execute. It’s processing code might look something like this:
- Verify the new user is for the same Customer as the Rule
- Check if conditions pass (arbitrarily configured business logic)
- If conditions pass, then do the action
Similarly to the HTML example, you can also create many Rules for the same broadcast. Let’s say that in addition to the custom email, we also want to send the customer a custom SMS Message. We can do that easily. Let’s simply add an additional Rule:
When a user is created, if conditions are true then send custom email
AND
When a user is created, if conditions are true then send custom text
You can add as many rules as you would like to be triggered. One thing to note, I would suggest that you process these rules in a background job on your server. When you add many rules, things can become computationally expensive. You don’t want your user waiting when they are signing up for all this stuff to happen!
The Rule System Final Thoughts
Similarly to the first two building blocks, the ultimate goal is to move the responsibilities of software engineers to the configuration team. The Rule system takes things one step further by actually allowing the configurers to orchestrate basic workflows.
Before, a customer request such as “Can we send a tweet every time an order is successful” can be a difficult and expensive request to fulfill by software engineering. Now, all the software engineering team needs to do is add a new action to the Rule System. Once that is completed, every customer going forward can be taken care of by the configuration team if they need something to be tweeted.
Over time, you will create many “hooks” (or triggers) throughout your application so that the configuration team can add more functionality at the right time for customers. Once again, the great thing about this system is that once you have a good library of actions, the configuration team can take care of many customers without the developers ever hearing about those requirements. Functionality can be added, removed and modified through the rule system without ever changing a single line of code for existing actions and triggers.